Getting Started with Meraki API using Python Part 6 - Functions
Welcome to Part 6 of this Getting Started series!
In this part, we will look at functions.
If you missed the earlier parts of our series, please make sure you go back to review part one before diving in.
Functions are a very important concept in programming. They allow you to reuse code so you don’t have to write the same code every time.
For example, many operations with the Meraki Dashboard require you to have the organization ID and network ID.
Here is an API endpoint to claim devices into a network which requires the network ID.

Here is an API endpoint to claim your order and add to the organization inventory.
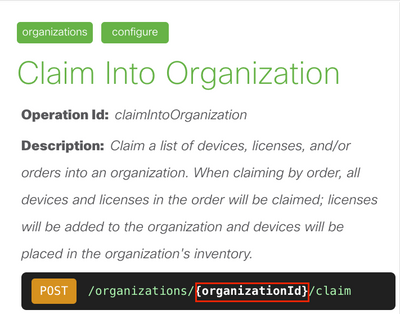
So rather than rewrite the code for getting the network ID and organization ID every time we need those values, we can instead create a function for it and call on the function anytime we need the ID.
Let’s get started on how to create Python functions!
If you have followed all the parts in this series so far, you should now be familiar with retrieving a list of organizations and filtering the list for particular organization parameters. Let’s have a look at the same code but now as a function.
Here is a function for getting the organization ID of DevNet Sandbox:
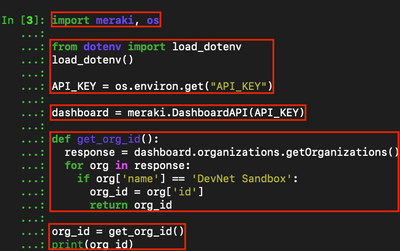
Let’s analyze the code line by line.
This statement imports the meraki and os python libraries.
import meraki,os
This block of code allows the script to read the .env file.
from dotenv import load_dotenv
load_dotenv()
This statement sets the API key for the meraki library functions imported.
dashboard = meraki.DashboardAPI(API_KEY)
This block of code is the function.
def get_org_id():
response = dashboard.organizations.getOrganizations()
for org in response:
if org[‘name’] == ‘DevNet Sandbox’:
org_id = org[‘id’]
return org_id
Let us focus on this block of code in a bit more detail. First, we define a function using def and the name of the function. Here we call it get_org_id().
def get_org_id():
Then we do a GET request to retrieve a list of organizations. This line is indented to tell the script that this is run within the function block.
response = dashboard.organizations.getOrganizations()
Then we use a for loop, as we have seen before to iterate through the list of organizations stored in response and look for the specific organization name ‘DevNet Sandbox’ and then if the name matches, store its organization ID in a variable org_id.
for org in response:
if org[‘name’] == ‘DevNet Sandbox’:
org_id = org[‘id’]
Then we return the value of the organization ID whenever this function is called using the return statement.
return org_id
How do you call a function?
Here is the statement which does that.
org_id = get_org_id()
We create a variable called org_id and then call our function get_org_id. Remember that the function returns the value of the organization ID, which gets stored in the variable org_id. Now that we have the value of the organization ID stored in the variable, all we need to do is to print the variable!
print(org_id)
Let us look at one more example.
Now that we have the organization ID, what can we do with it? The next step would be to get the network ID of a network within the organization, we can then configure firewall rules, claim devices etc for the network with the network ID. So let’s create a function for that.

Let’s analyze this code block.
First, we create a function called get_net:
def get_net(org_id):
Within the braces, we have the parameter org_id. We can pass values to function code blocks using parameters within braces. In this case, we are sending the organization ID, which the function needs in order to retrieve a list of networks in the organization.
Next, we take the org_id parameter passed to the function and then store it in a variable called organization_id.
organization_id = org_id
Then we do a GET request for a list of networks in the organization.
response = dashboard.organizations.getOrganizationNetworks(
organization_id, total_pages='all')
We use a for loop to iterate through the list of networks stored in response, and if the network name matches ‘DevNet Sandbox ALWAYS ON’, then we print the network name and ID.
for net in response:
if net['name'] == 'DevNet Sandbox ALWAYS ON':
print(net['name'], '->', net['id'])
Then we call the function:
get_net(org_id)
We send the value stored in org_id to the function when we call it.
The output is given below:
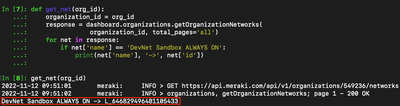
That’s it for this post! I will leave it here to let you try it out yourself!
Also don’t forget to subscribe to Getting Started to get notified on the next post!
Meraki - To do something with soul, creativity or love.