Hi Everyone! Welcome to Part 5 of a series of posts to get you started with Meraki APIs using Python!
In this post, we will learn about the differences in outputs we get when using Meraki library v/s Requests library, and then we will learn how to further manipulate the requests response and convert it into a usable format. Let's Begin!
In Part 4, we discussed what the request library is, how to install it, and then finally ran an API call using the same and received a response for it. We ended the post with a question of whether or not you were able to notice anything different. Let's take a look at it.
Here is the response when using Meraki library:
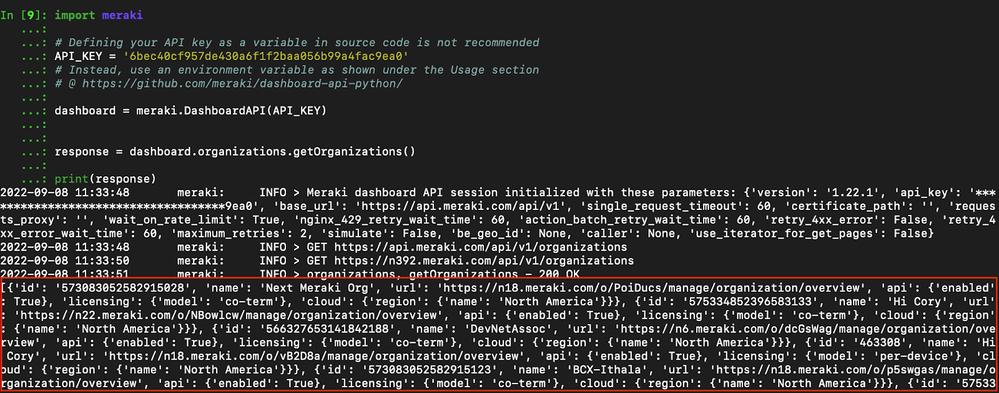
And, here is the response when using the request library:
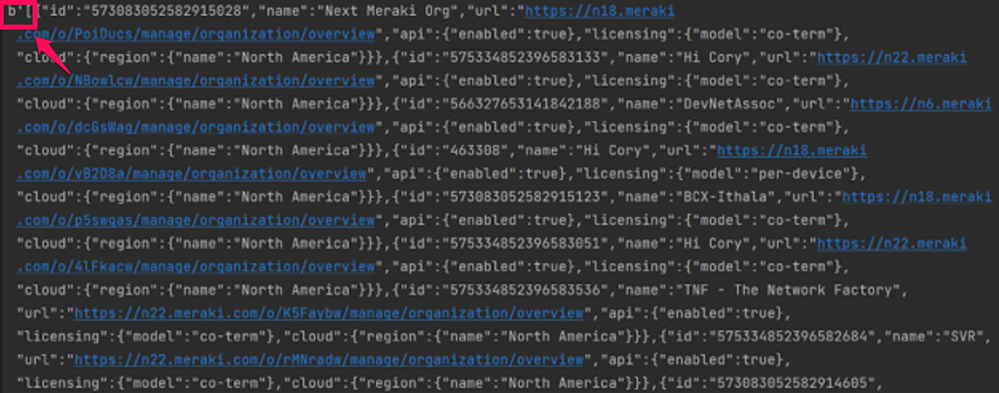
Look at the highlighted little block above in red. That's the difference! The “b” represents literal bytes. You can also use the type() method to verify. I know it's very subtle, but that explains why it is important to understand the data you get back from an API call using whichever library you choose. Then you can manipulate the data in any way you want so you can make use of it later in the code.
When you use the Meraki library, you can directly print the response variable because it by default will give you data as a list which you can iterate through and get the required values from easily. On the other end, you can't just print the response variable directly when using the request library because it gives you only a status code in return like 200 OK or something, not the actual data. Furthermore, as we did in the last part, response.text will return the actual output in “string” and then after encoding it to UTF8 will give us data in “raw bytes”. Now from a visual perspective, they both look the same, but from a practical standpoint we cannot use either of those data further in the code to tap into specific values like org ID, or name, or anything else for that matter.
So, how can we manipulate raw bytes or string data and convert it into a usable format?
The answer is the “json()” method. The requests library actually has a built-in json() method that can be used to parse the stream of response data and convert it into JSON format. You simply do this by adding .json() at the end of your response as follows:
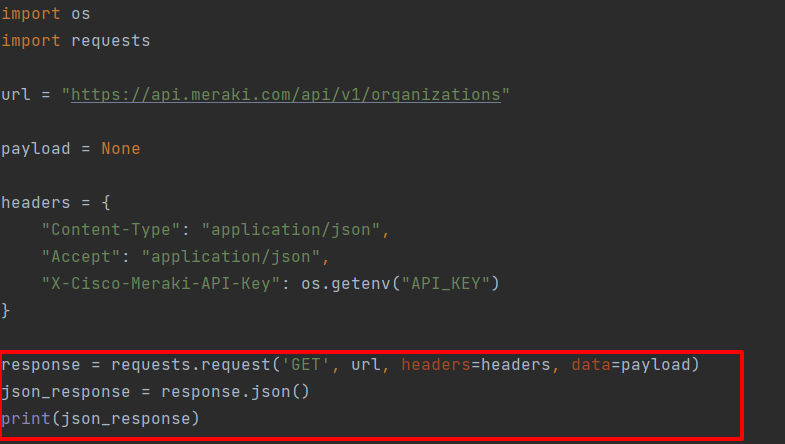
Now, you can directly print the json_response variable which in our case now would be a list of JSON objects where each object will represent one individual organization as follows:
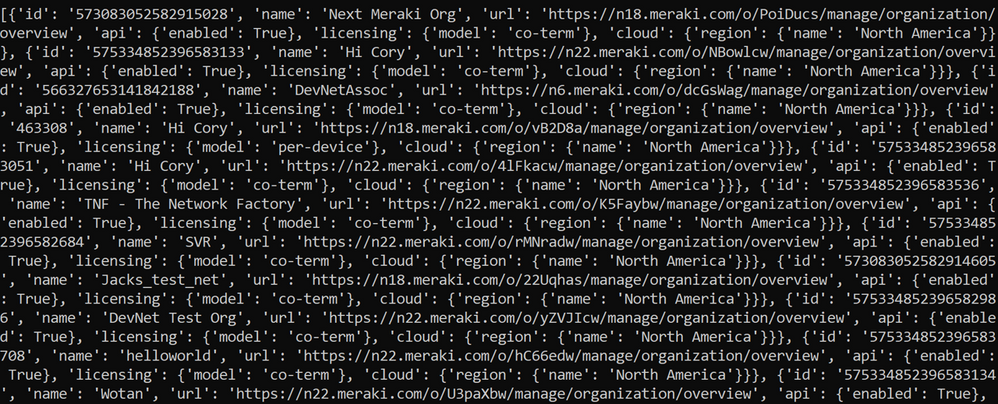
Alright! Now you may use the response variable within an if-else statement to see if the API call went through (200 OK) or if it came back with an error so you can handle it gracefully. Then you can use the converted JSON data in form of json_response variable for rest of your code. So after a little tweaking we now have the same usable output similar to what we got from the Meraki library.
But does anyone feel that the output is not easily readable or structured enough?
Well, if you answered yes then I am glad I am not the only one wild enough to even attempt to read this output. After all, it's supposed to be JSON which is super easy to read, right?
To make the output look a bit better, you can import the JSON library which comes pre-installed as a standard library with Python so there is no extra installation to do. There are two methods or functions within this JSON library called loads() and dumps() which can be used to load a string as an input and dump it as a JSON output respectively. The easiest way to remember this is that the “s” at the end of loads() and dumps() represent a string as an input. You won't have to use the loads() method because it's exactly the same as adding .json() at the end of an API call. But, you will use the dumps() method to make the output readable as follows:
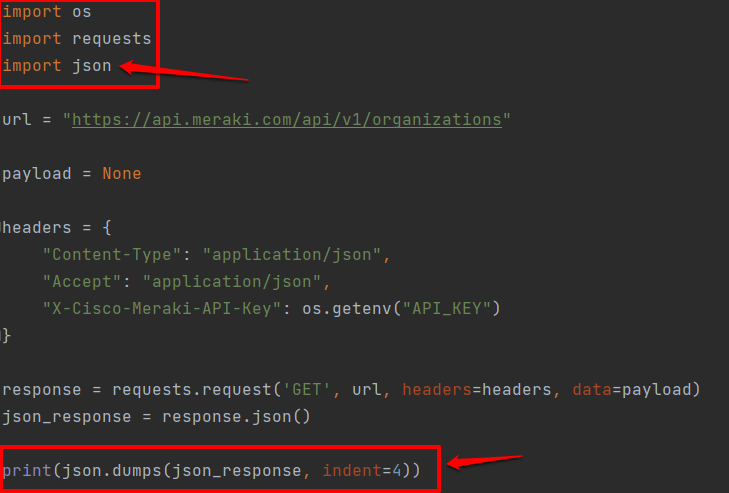
Start by importing the JSON library by using import json in the beginning. Then you can print the actual data by using json.dumps(json_response, indent=4). The indent parameter adds the indent required for JSON data to look readable, and we chose the number 4 because, as per the best practices, there should be 4 spaces for any indent in Python.
In summary, if you just care about getting a usable output from an API call, then the .json() method will do the job. However, if you care about that output to look pretty and readable, then you gotta manipulate the data a bit more using json.dumps() method.
The output will now look much prettier and more readable as follows:
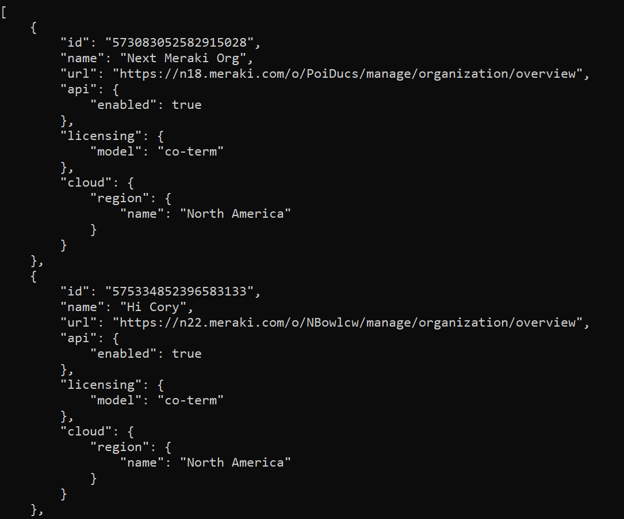
Remember the response variable from the most recent code above is still a list of JSON objects just like before, but now you can print it in a more readable manner. This means you can perform the same loops and if-else operations to tap into something specific as we did back in Part 3 to get a list of org ID, name, or any other specific value from that data stored in the response variable.
The question now becomes which library would you choose: Meraki or Requests?
The answer is it depends. It's really up to you to choose whichever library you feel most comfortable with. The most important thing to remember is knowing how to find out what kind of data you are receiving and then how to manipulate it in a way that is useful for the rest of the code. As we proceed in this journey, I would highly encourage you all to play around with both libraries and find out which one makes it easier for you to compile and run an API call.
We will sign off here for now, but look out for Part 6 in this series to continue the journey further!
If you have any questions, don’t hesitate to ask us below. We welcome your feedback as well. Also don’t forget to subscribe to Getting Started to get notified on the next post!